Main page
Table of contents
Setting up MinGW-w64 11.2
- Click this link to download MinGW-w64 11.2
- Unzip it to the C drive
- Add this path: "C:\mingw64\bin" to the PATH environment variable
- Note. You can open the environment variable dialog by using this command in CMD: rundll32 sysdm.cpl,EditEnvironmentVariables
- Open a new CMD and type this command: "g++ --version" to check that it set up
- If you want to try another version of MinGW-w64 use this link: https://winlibs.com/
Setting up CMake
- Download the CMake installer: https://cmake.org/download/
- Install CMake with the default setting
- Open a new CMD and type this command: "cmake --version" to check that it set up
- I use CMake 3.27.7
Build "Hello, World" in plain C++ from CMD
- Create an empty folder for a project, for example: hello-world
- Create a CMakeLists.txt file with the following contents:
cmake_minimum_required(VERSION 3.20)
project(hello-cmake)
add_executable(app)
target_sources(app PRIVATE main.cpp)
target_link_options(app PRIVATE -static)
Create a main.cpp file with the following contents:
#include <iostream>
int main()
{
std::cout << "Hello world!" << std::endl;
return 0;
}
Open CMD in the project folder
Type the following command:
cmake -G "MinGW Makefiles" -S . -B dist
Go to the "dist" folder
cd dist
Build the application using this command:
cmake --build .
Run the application using this command:
app
Writting the batch files to config and build
- Create a "hello world" example above
- Create a "config.bat" file
- Add the following contents to the file:
cmake -G "MinGW Makefiles" -S . -B dist
Create a "build.bat" the following contents to the file:
cd dist
cmake --build .
Type the "config" command and "build" to configure and build the applcation
Run the application using this command:
app
Type "cmake --build ." to rebuild the application
Setting up SDL 3.1.3 in CMD
- Click this link: SDL3-devel-3.1.3-mingw.zip to download prebuild SDL 3.1.3
- Note. If you want to try another version of SDL go here: https://github.com/libsdl-org/SDL/releases/
- Unzip the archive somewhere. For example, I've unzipped it to "E:\libs\sdl-3.1.3-prefix\win"
- Add this path: "E:\libs\sdl-3.1.3-prefix\win\bin" to the PATH environment variable. This folder contains SDL3.dll
- Create an empty folder with the name "hello-sdl3"
- Create a CMakeLists.txt file inside of the "hello-sdl3" folder with the following contents:
cmake_minimum_required(VERSION 3.20)
project(hello-sdl3)
add_executable(app) # WIN32 - hide the console like this add_executable(app WIN32)
target_sources(app
PRIVATE
main.cpp
)
find_package(SDL3)
target_link_libraries(app PRIVATE SDL3::SDL3)
target_compile_definitions(app PRIVATE SDL_MAIN_USE_CALLBACKS)
target_link_options(app PRIVATE -static)
Create a main.cpp file and copy the official example to this file that you can find inside of the source of SDL3 here "examples/template.c":
/*
* This example code $WHAT_IT_DOES.
*
* This code is public domain. Feel free to use it for any purpose!
*/
#define SDL_MAIN_USE_CALLBACKS 1 /* use the callbacks instead of main() */
#include <SDL3/SDL.h>
#include <SDL3/SDL_main.h>
/* We will use this renderer to draw into this window every frame. */
static SDL_Window *window = NULL;
static SDL_Renderer *renderer = NULL;
/* This function runs once at startup. */
SDL_AppResult SDL_AppInit(void **appstate, int argc, char *argv[])
{
SDL_SetAppMetadata("Example HUMAN READABLE NAME", "1.0", "com.example.CATEGORY-NAME");
if (!SDL_Init(SDL_INIT_VIDEO)) {
SDL_Log("Couldn't initialize SDL: %s", SDL_GetError());
return SDL_APP_FAILURE;
}
if (!SDL_CreateWindowAndRenderer("examples/CATEGORY/NAME", 640, 480, 0, &window, &renderer)) {
SDL_Log("Couldn't create window/renderer: %s", SDL_GetError());
return SDL_APP_FAILURE;
}
return SDL_APP_CONTINUE; /* carry on with the program! */
}
/* This function runs when a new event (mouse input, keypresses, etc) occurs. */
SDL_AppResult SDL_AppEvent(void *appstate, SDL_Event *event)
{
if (event->type == SDL_EVENT_QUIT) {
return SDL_APP_SUCCESS; /* end the program, reporting success to the OS. */
}
return SDL_APP_CONTINUE; /* carry on with the program! */
}
/* This function runs once per frame, and is the heart of the program. */
SDL_AppResult SDL_AppIterate(void *appstate)
{
return SDL_APP_CONTINUE; /* carry on with the program! */
}
/* This function runs once at shutdown. */
void SDL_AppQuit(void *appstate, SDL_AppResult result)
{
/* SDL will clean up the window/renderer for us. */
}
Copy and paste this command in CMD to configure:
cmake -G "MinGW Makefiles" -S . -B dist -DSDL3_DIR=E:/libs/sdl-3.1.3-prefix/win/lib/cmake/SDL3
Go to the "dist" folder
cd dist
Build the application using this command:
cmake --build .
Run the application using this command:
app
Note. You don't see a window because it is just a template
Setting up Qt Creator with Qt 6.6.3
- Use the offical Qt installer and check this flags:
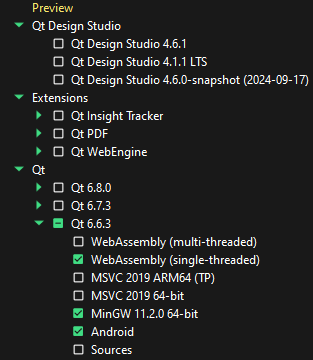
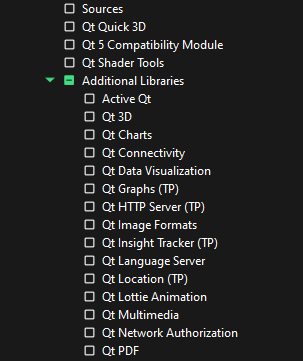
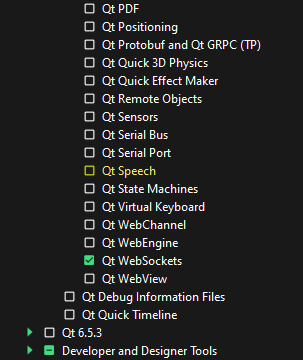
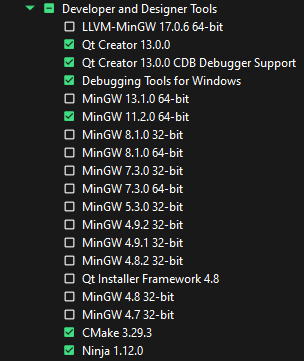
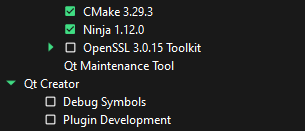
Build "Hello, World" in plain C++ from Qt Creator
- Run Qt Creator
- Go to "File" > "New Project..." > "Non-Qt Project" > "Plain C++ Application" and so on. The CMakeLists.txt file and the "main.cpp" file will be created
- Click on the gree triangle button in the left botton corner to buid and run the application or press Ctrl+R
- You will see the "Hello World!" text in the "Application Output" window
- You can set breakpoint and click on the green triangle button with a bug. This is the next button after the green triangle
Setting up GLAD
- Let's setup GLAD to define OpenGL functions. Google "glad online opengl generator". You will find this link: https://glad.dav1d.de/
- In the "API > gl" select the OpenGL API version what do you want. I prefer to use "Version 2.1" because it is very similar to OpenGL ES 2.0 that is enough for me
- Scroll to the bottom of the page and click on the "Generate" button
- Click on the "glad.zip" archive. Unzip in somewhere. For example: "E:\libs\glad-2.0.6"
Setting up SDL3 and GLAD in Qt Creator
- There are two ways to create a CMake project in Qt Creator. The first way: "File" > "New Project..." > "Non-Qt Project" > "Plain C++ Application" and so on. The CMakeLists.txt file and the "main.cpp" file will be created
- The second way. You can manually create the "CMakeLists.txt" and "main.cpp" file. Go to the menu of Qt Creator and choose: "File" > "Open File or Project..."
- Create a CMake project in Qt Creator using the first or the second way above
- Replace the contants of CMakeLists.txt with this one:
cmake_minimum_required(VERSION 3.20)
project(opengl-example-in-sdl3)
add_executable(app) # WIN32 - hide the console like this add_executable(app WIN32)
target_include_directories(app PRIVATE E:/libs/glad-2.0.6/include)
target_include_directories(app PRIVATE E:/libs/glm-1.0.1-light)
target_sources(app
PRIVATE
E:/libs/glad-2.0.6/src/glad.c
main.cpp
)
find_package(SDL3)
target_link_libraries(app PRIVATE SDL3::SDL3)
target_compile_definitions(app PRIVATE SDL_MAIN_USE_CALLBACKS)
target_link_options(app PRIVATE -static)
Replace the main.cpp file contains with this one:
#include <SDL3/SDL.h>
#include <SDL3/SDL_main.h>
#include <glad/glad.h>
#include <iostream>
struct AppContext
{
SDL_Window *window;
SDL_GLContext glContext;
SDL_AppResult appQuit = SDL_APP_CONTINUE;
};
SDL_AppResult SDL_Fail()
{
SDL_LogError(SDL_LOG_CATEGORY_CUSTOM, "Error %s", SDL_GetError());
return SDL_APP_FAILURE;
}
SDL_AppResult SDL_AppInit(void **appstate, int argc, char *argv[])
{
// Init the library, here we make a window so we only need the Video capabilities
if (!SDL_Init(SDL_INIT_VIDEO))
{
std::cout << "hello" << std::endl;
return SDL_Fail();
}
SDL_GL_SetAttribute(SDL_GL_MULTISAMPLEBUFFERS, 1); // Enable MULTISAMPLE
SDL_GL_SetAttribute(SDL_GL_MULTISAMPLESAMPLES, 2); // can be 2, 4, 8 or 16
// Create a window
SDL_Window *window = SDL_CreateWindow("SDL3, OpenGL 2.1, C++", 350, 350,
SDL_WINDOW_OPENGL); // | SDL_WINDOW_RESIZABLE
if (!window)
{
return SDL_Fail();
}
SDL_GLContext glContext = SDL_GL_CreateContext(window);
if (!glContext)
{
return SDL_Fail();
}
if (!gladLoadGL())
{
std::cout << "Failed to load OpenGL functions" << std::endl;
return SDL_APP_FAILURE;
}
glClearColor(0.2f, 0.5f, 0.3f, 1.f);
SDL_ShowWindow(window);
// Set up the application data
*appstate = new AppContext {
window,
glContext,
};
return SDL_APP_CONTINUE;
}
SDL_AppResult SDL_AppEvent(void *appstate, SDL_Event *event)
{
auto *app = (AppContext *)appstate;
switch (event->type)
{
case SDL_EVENT_QUIT:
{
app->appQuit = SDL_APP_SUCCESS;
break;
}
default:
{
break;
}
}
return SDL_APP_CONTINUE;
}
SDL_AppResult SDL_AppIterate(void *appstate)
{
auto *app = (AppContext *)appstate;
glClear(GL_COLOR_BUFFER_BIT);
SDL_GL_SwapWindow(app->window);
return app->appQuit;
}
void SDL_AppQuit(void *appstate, SDL_AppResult result)
{
auto *app = (AppContext *)appstate;
if (app)
{
SDL_GL_DestroyContext(app->glContext);
SDL_DestroyWindow(app->window);
delete app;
}
SDL_Quit();
}
Click on the "Project" tab on the left panel
Add this pair key/value to the "Initial Configuration" table:
Key: SDL3_DIR
Value: E:\libs\sdl-3.1.3-prefix\win\lib\cmake\SDL3
Click on the "Re-configure with Initial Parameters" button below of the table
Click on the "Edit" tab on the left panel to return to source code of the project
Click on the gree triangle button in the left botton corner to buid and run the application or press Ctrl+R
You will see a window with a green background